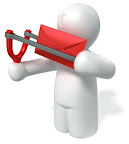
In this example, we send messages with SMTP. We receive, we read or we delete messages with POP3. We also add and display attachments.
Components used in this example
- Zend_Mail
- Zend_Mail_Exception
- Zend_Mail_Storage_Pop3
- Zend_Mail_Transport_Smtp
- Zend_Mime
- Zend_Mime_Part
- Zend_Service_ReCaptcha
- Zend_Validate_EmailAddress
Working with SMTP and POP3
class MyMail
{
const PASSWORD = 'data/mail/password.php';
const BASE_KEY = 'data/mail/generate-key.php';
const RECAPTCHA_KEYS = 'data/mail/recaptcha-keys.php';
const SUBJECT = 'Your key is ';
const BODY = "
Please, delete this email if you believe you received it by mistake. Sorry.
请,删除此电子邮件,如果你相信你收到失误。对不起。
Por favor, elimine este correo electrónico si usted cree que usted recibió por error. Perdón.
もし誤って受け取ったと信じてください、このメールを削除します。残念に思う。
S'il vous plaît, supprimer ce message si vous croyez que vous l'avez reçu par erreur. Désolé.
Por favor, apague este e-mail se você acredita que o recebeu por engano. Desculpe.
Bitte löschen Sie diese E-Mail, wenn Sie glauben, sie erhalten hat, aus Versehen. Bekümmert.
يرجى حذف هذا البريد الإلكتروني إذا كنت تعتقد أنها وردت عن طريق الخطأ. آسف.
Пожалуйста, удалите это сообщение, если Вы считаете, что получили его по ошибке. Извините.
만약 당신이 실수로 그것을받은 믿을 제발,이 이메일을 삭제할 수있습니다. 죄송합니다.
John & Jane (ZfEx)
";
Processing the messages- We get the data to create and send a message, or the action to perform on the mailbox.
- We create the Captcha that is required to send a message.
- We instantiate the POP3 connection.
- We get the list of messages of John or Jane.
- We process the main action.
- If we catch an exception, we return the error message.
public function process()
{
// We get the data to create and send a message,
// or the action to perform on the mailbox.
list($from, $yourEmail, $yourName, $yourKey,
$addTo1, $email1, $addTo2, $email2, $subject, $body, $challenge, $response,
$id, $action, $verbose, $submit) = $this->_getParameters();
$messages = array();
$attachments = array();
try {
// We create the Captcha that is required to send a message.
list($pubKey, $privKey) = include self::RECAPTCHA_KEYS;
$recaptcha = new Zend_Service_ReCaptcha($pubKey, $privKey);
$recaptcha->setOption('theme', 'white');
// We instantiate the POP3 connection.
$storage = New MyPop3($from, $verbose);
// We get the list of messages of John or Jane.
$messages = $storage->getMessages($yourEmail);
// We process the main action.
if ($submit == 'Send me my key') {
$result = $this->_sendKey($from, $yourEmail, $yourName);
} else if ($submit == 'Validate') {
if (empty($yourEmail)) {
$result = 'Please, enter a valid email address!';
} else if ($yourKey != self::getKey($yourEmail)) {
$result =
'Your email and key combination is invalid!' .
' Please, correct them.';
} else {
$result = 'Your email and key are valid.';
}
} else if ($yourEmail and $yourKey != self::getKey($yourEmail)) {
$result =
'Your email and key combination is invalid or your key has expired!' .
' Please, correct them or get a new key.';
} else if ($submit == 'Send') {
$result = $this->_send($from, $yourEmail, $yourName,
$addTo1, $email1, $addTo2, $email2, $subject, $body,
$challenge, $response, $verbose, $recaptcha);
} else if ($submit == 'Submit'){
list($messages, $result, $attachments) =
$this->_submit($id, $action, $storage, $messages);
} else {
$result =
"You are logged on as $from." .
' Please fill the form to send a message or check messages.';
}
} catch (Exception $e) {
// If we catch an exception, we return the error message.
$result = $e->getMessage();
}
return array($from, $yourEmail, $yourName, $yourKey,
$addTo1, $email1, $addTo2, $email2, $subject, $body,
$id, $action, $verbose, $messages, $result, $attachments, $recaptcha);
}
Extraction of the parameters from the POST request
private function _getParameters()
{
$from = isset($_POST['from'])? $_POST['from'] : 'john@micmap.org';
$yourEmail = isset($_POST['your-email'])? $_POST['your-email'] : '';
$validate = new Zend_Validate_EmailAddress();
$validate->isValid($yourEmail) or $yourEmail = '';
$yourName = isset($_POST['your-name'])? $_POST['your-name'] : '';
$yourKey = isset($_POST['your-key'])? $_POST['your-key'] : '';
$addTo1 = (isset($_POST['add-to-1']) and isset(MySmtp::$addTo[$_POST['add-to-1']]))?
$_POST['add-to-1'] : 'addTo';
$email1 = (isset($_POST['email1']) and ($_POST['email1'] == $yourEmail or
isset(MySmtp::$names[$_POST['email1']])))? $_POST['email1'] : null;
$addTo2 = (isset($_POST['add-to-2']) and isset(MySmtp::$addTo[$_POST['add-to-2']]))?
$_POST['add-to-2'] : 'addTo';
$email2 = (isset($_POST['email2']) and ($_POST['email2'] == $yourEmail or
isset(MySmtp::$names[$_POST['email2']])))? $_POST['email2'] : null;
$subject = isset($_POST['subject'])? $_POST['subject'] : '';
$body = isset($_POST['body'])? $_POST['body'] : '';
$challenge = isset($_POST['recaptcha_challenge_field'])?
$_POST['recaptcha_challenge_field'] : null;
$response = isset($_POST['recaptcha_response_field'])?
$_POST['recaptcha_response_field'] : null;
$id = isset($_POST['id'])? $_POST['id'] : null;
$action = isset($_POST['action'])? $_POST['action'] : null;
$verbose = empty($_POST)? 0 : !empty($_POST['verbose']);
$submit = isset($_POST['submit'])? $_POST['submit'] : null;
return array($from, $yourEmail, $yourName, $yourKey,
$addTo1, $email1, $addTo2, $email2, $subject, $body, $challenge, $response,
$id, $action, $verbose, $submit);
}
Sending a key to an external email address
private function _sendKey($from, $yourEmail, $yourName)
{
if ($yourEmail) {
$smtp = new MySmtp($from);
$yourSubject = self::SUBJECT . self::getKey($yourEmail);
$smtp->shoot($yourEmail, $yourName, $yourSubject, self::BODY,
'addTo', $yourEmail);
$result = 'Your key was sent to your email.';
} else {
$result = 'Please, enter a valid email address!';
}
return $result;
}
Sending a message
private function _send($from, $yourEmail, $yourName,
$addTo1, $email1, $addTo2, $email2, $subject, $body, $challenge, $response,
$verbose, $recaptcha)
{
if ($recaptcha->verify($challenge, $response)->isValid()) {
$smtp = new MySmtp($from);
$smtp->shoot($yourEmail, $yourName, $subject, $body,
$addTo1, $email1, $addTo2, $email2);
$result = $verbose? $smtp : 'You message was sent.';
} else {
$result =
'The reCAPTCHA wasn\'t entered correctly! Go back and try it again.' .
' (Note that you have to reattach files that were previously attached.)';
}
return $result;
}
Performing an action on a mailbox
private function _submit($id, $action, $storage, $messages)
{
$attachments = array();
switch ($action) {
case 'countMessages':
case 'getSize':
case 'getUniqueId':
$result = $storage->$action();
break;
case 'getMessage':
case 'getRawContent':
case 'getRawHeader':
$number = $storage->getNumberByUniqueId($id);
$result = $storage->$action($number);
break;
case 'readMessage':
$number = $storage->getNumberByUniqueId($id);
list($result, $attachments) = $storage->readMessage($number);
break;
case 'removeMessage':
$result = $storage->deleteMessage($id);
unset($messages[$id]);
$result = 'Your message removal request was sent.';
break;
default:
$result = 'The message list is refreshed';
}
return array($messages, $result, $attachments);
}
Creating a key to allow the use of an external email- The key is the CRC32 of the external email address and a base key. The base key is calculated from a private key. The base key has a lifespan of up to 9999 seconds.
public static function getKey($yourEmail)
{
// The key is the CRC32 of the external email address and a base key.
// The base key is calculated from a private key.
// The base key has a lifespan of up to 9999 seconds.
return hash('crc32', $yourEmail . '-' . include self::BASE_KEY);
}
}
No comments:
Post a Comment